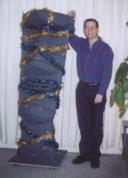
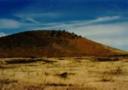
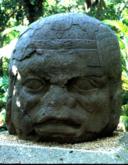
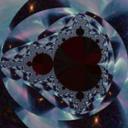
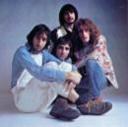
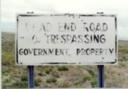
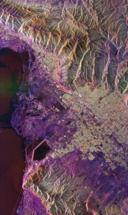
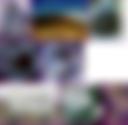
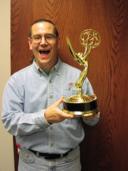
|
Direct3D Graphics Pipeline Code Samples
This code has been made in preparation for my book on Direct3D. The web versions of these
samples will lag the actual samples for the book until the book is
published. Simply put, its more important for me to finish the book
than it is to keep this web site in synch with the latest changes to
the samples. Once the book has been published, the most up-to-date
versions of all the samples will be available at a web site through
the publisher.
Notes on the samples. Useful tools.
You can also browse individual files in
the samples.
Name | Description | Download Links |
GingerBread |
Based on the minimal application, this
program computes a chaotic orbit in 2D and displays the orbit as color
coded points. The chaotic nature of the orbit makes each painting of
the window's client area slightly different, appearing animated. This
can be a very simple way to experiment with an animated effect without
coding timers or using multiple threads. Drag the window size handles
and watch.
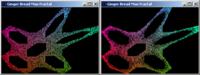
|
gingerbread.zip 1.1
gingerbread.exe |
Minimal |
Source code for a minimal well-behaved Direct3D8 application that
draws a single triangle in response to WM_PAINT
messages.
|
minimal.cpp |
rt |
No, not "yet another set of wrapper classes around DirectX
interfaces". Instead, this provides some generic utilities that are
of use when writing Win32 C++ programs. This library has evolved from
my personal efforts. Things that I find myself needing repeatedly go
into this library. The library includes a mechanism for mapping
unexpected COM failed HRESULTs to C++ exceptions, C++ standard library
string classes adapted to TCHAR, and a few miscellaneous items.
19-Mar-2001: Use DXGetErrorString8 instead of
D3DXGetErrorString. Include code fragment that generates the HRESULT
in the failure message. (Suggested by Andrew Vesper.) Add uuid()
support for D3DX and X file interfaces.
|
rt.zip 1.2 |
rt_All |
Builds all the SDK samples in one workspace.
Unzip the archive into the Direct3D sample directory. You will get two
files: a VC++6 workspace a project. The project is a utility project
that is dependent on all the Direct3D samples and tutorials shipped in
the DirectX 8.0 SDK. The workspace contains the "rt_All" project
and all the SDK sample projects. Open up the workspace, select the
"rt_All" project, select a build configuration, and then build the
project to get all the SDK samples built. Because this uses the
project files as supplied in the SDK it doesn't build a library of the
shared code, so it can take some time as each sample will compile its
own copy of the shared framework.
Note that this workspace doesn't include any sample or example
programs I've written myself, only the Direct3D 8 samples distributed
with the DX8 SDK.
Unpack into %DX8SDK%\samples\multimedia\Direct3D.
|
rt_All.zip 1.3 |
rt_Apprentice |
What's an application apprentice? Well its a code generator that's
not quite smart enough to be called an Application Wizard, in MSVC
parlance. The apprentice is a code generator, just like an AppWizard,
but its just an extremely dumb code generator. All it knows how to do
is make a new Direct3D sample from a template with your entered project
name substituted in a few places.
Included are templates for building a "minimal" D3D application as
either a console application of a Win32 application, a screen saver
based on CD3DScreenSaver, or a Visual Basic application based on the
VB SDK framework.
rt_Apprentice.hta is an HTML application (requires IE5
or later) that prompts you to select the application type. After
selecting the application type you are prompted for the name of the
new project and its location. The project name gets substituted into
the template for the project type into new files for the project. Be
sure to use a valid identifier for the project name (i.e. no spaces or
other characters prohibited in identifiers) as the project name is
substituted into pieces of code as an identifier in some instances.
The generates projects a 'blank' and do nothing useful really. You
must modify the generated code to make the project do something
interesting, but it does get the boiler plate code out of the
way.
Unpack anywhere.
Note: HTML applications require the presence of IE5 or later.
If you don't have IE5 installed on your computer, then you can carry
out the apprentice's actions manually by copying the appropriate
template directory to your new project directory and replacing
$$BLANK$$ with your project name in the template files.
|
rt_Apprentice.zip 2.0 |
rt_D3DXSphere |
Sample based on D3DFrame, the boiler plate classes that Microsoft
uses for their DirectX SDK sample programs. This sample was started
by using the "New Sample Apprentice" below to generate a blank sample
and then add demonstrations of D3DXCreateBox, D3DXCreateCylinder,
D3DXCreateSphere, D3DXCreateTeapot and D3DXCreateTorus. Interactive
control over the detail of the generated mesh is provided through the
arrow keys on the keyboard. Unpack into your Direct3D samples
directory (%DX8SDK%\samples\multimedia\Direct3D). It will create a
subdirectory called rt_D3DXSphere containing the sample.
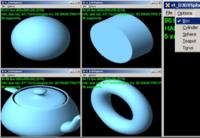
Demonstrates:
device initialization, simple viewing, simple lighting, Z buffering,
D3DXCreateBox, D3DXCreateCylinder, D3DXCreateSphere, D3DXCreateTeapot,
D3DXCreateTorus D3DXMatrixLookAtLH, D3DXMatrixOrthoLH, ID3DXMesh,
ID3DXBuffer, ID3DXMesh::OptimizeInplace.
Unpack into %DX8SDK%\samples\multimedia\Direct3D.
|
rt_D3DXSphere.zip 1.2
|
rt_D3DXSprite |
Demonstrates drawing 2D sprites with D3DX. You can display a
sprite tiling the entire surface of the window and a dynamically
rotating collection of sprites. Use the arrow keys to increase or
decrease the number of dynamically rotating sprites.
Use the menus to change the sprites that are displayed each frame,
alter the color of the background, the sprite modulation color and the
sprite texture's color key. You can also changing mirroring options
for the tiled sprite.
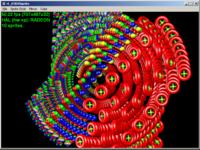
Demonstrates: ID3DXSprite, D3DXCreateTextureFromFileEx
Unpack into %DX8SDK%\samples\multimedia\Direct3D.
Try loading "donuts1.bmp" from the
%DX8SDK%\samples\multimedia\Media directory to see color keying
effects.
|
rt_D3DXSprite.zip 1.0 |
rt_FrameBuffer |
Visualizes frame buffer processing: alpha test, stencil/depth
test, stencil masking, stencil stippling, alpha blending (including
blend modes using destination alpha), multisample antialising,
multisample motion blur, multisample depth of field, dithering,
and color channel masking.
This sample makes use of destination alpha and stencil planes by
default. Your hardware may not support these. If you're getting a
dialog box saying that no suitable devices could be found and that the
reference rasterizer will be used instead, then try running the
program with the following command-line switches:
-nodest | Don't use destination alpha. |
-nostencil | Don't use stencil planes. |
Alpha blending screen
shots
Can be unpacked anywhere.
Note: This sample was created with the DX8.1 SDK
AppWizard.
Updated 05-Mar-2002: rt_FrameBuffer now validates
the blend modes used and disables any blending modes not supported by
the device. Background and diffuse colors now allow unassociated
alpha RGBA (i.e. R, G, B can be larger than A).
|
rt_FrameBuffer.zip 1.0 |
rt_Gamma |
Did you know that RGB(128, 128, 128) is not half as bright as
RGB(255, 255, 255)? Surprise! Welcome to the world of CRT monitors
and their non-linearities. LCD displays and printers also have
non-linearities in their color response. For CRTs, the non-linearity
can be modeled as a simple power function correlating intensity I with
CRT voltage V to the gamma power. The use of gamma in this formula is
why the process of compensating for the nonlinearity is called
"gamma correction".
Direct3D provides support for gamma correction directly in the
GetGammaRamp and SetGammaRamp methods of the device.
You can model a CRT's gamma as a single value for all phosphors or
you can measure separate values for the red, green and blue phosphors.
SetGammaRamp allows both techniques to be used. This program lets you
interactively measure the gamma characteristics of your monitor, both
as a single gamma value and as separate gamma values for red, green
and blue phosphors. It also provides a test rendering that you can
use to visualize the effects of gamma correction.
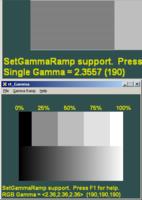
Demonstrates: D3DGAMMARAMP, SetGammaRamp(), technique for
interactively measuring a monitor's gamma value, when to gamma correct
yourself or let Direct3D do it, gamma test rendering, single gamma model,
RGB gammas model, manual gamma correction.
Unpack into %DX8SDK%\samples\multimedia\Direct3D.
|
rt_Gamma.zip 1.4 |
rt_GingerBread |
The gingerbread man fractal enhanced with D3DFrame. Unlike the
'minimal' style gingerbread man fractal above, this sample provides
you with much more control over the rendering with D3DFrame:
interactive device switching, a toggle from windowed mode to exclusive
mode, start/stop and single-step the animation, FPS counter and other
rendering statistics. Most of this comes from simply using D3DFrame's
implementation of CD3DApplication. Extending CD3DApplication to
provide more GUI elements is as easy as adding some menu items and a
command handler.
You can watch
the its chaotic orbit in the plane as diffuse points or textured
points. The points are rendered with alpha blending to allow them to
accumulate to a dense image. The standard color chooser dialog is
augmented with an alpha channel edit control, letting you edit all the
colors used in the sample. On-screen help, bounding box toggle, view
animation, status display toggle, foreground and background color
editing.
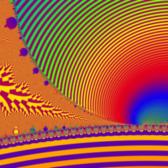
Demonstrates: diffuse colored points, texture creation, 2D texture
coordinate generation, textured points, single stage texture setup,
RGBA color picker with ::ChooseColor, accumulating renderings in the
back buffer, locking and filling vertex buffers, world transformation,
simple camera animation, IDirect3DVertexBuffer8, DrawPrimitive,
DrawPrimitiveUP, ValidateDevice.
Unpack into %DX8SDK%\samples\multimedia\Direct3D.
Just for fun, compare your HAL device to refrast on the same
settings. Are they the same?
|
rt_GingerBread.zip
1.2 |
rt_Lighting |
Visualizes lighting and material parameters and render states:
D3DLIGHT8 , D3DMATERIAL8 , RS Ambient,
RS Color Vertex, RS Lighting, RS Local Viewer, RS Normalize Normals,
RS Ambient Material Source, RS Diffuse Material Source, RS Specular
Material Source, RS Ambient Material Source, RS Specular Enable,
RS Shade Mode, and RS Fill Mode.
Simple dialogs let you change all the relevant material and light
parameters and visualize their results on the Utah teapot.
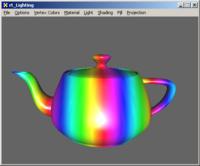
Can be unpacked anywhere.
Note: This sample was created with the DX8.1 SDK
AppWizard.
|
rt_Lighting.zip 1.0 |
rt_meshenum |
A program to dump out the contents of an ID3DXMesh object as
returned by the D3DXLoadMeshFromX function. Shows you how to get at
the face, index and vertex information for an ID3DXMesh by printing
out information about the mesh to the debug output stream.
28-Feb-2001: Now uses the display format instead
of x8r8g8b8 for the back buffer format that caused the CreateDevice
to fail. Added workaround for VC++6 template weakness (see code
comment in dump.cpp). Added a big comment at the top of dump.cpp
explaining the data organization of a mesh.
19-Mar-2001: Fixed some ANSI/UNICODE build problems.
|
rt_meshenum.zip 1.4 |
rt_Rasterize |
Demonstrates the rasterization related render states RS Last
Pixel, RS Edge Antialias, and RS Line Pattern. A scene consisting of
a screen-space grid and a world-space circle is rendered with lines.
To best observe the effect of RS Last Pixel, set the grid color to
something less than fully opaque and use a pixel zoom utility to
examine the intersections of the grid. The effect can also be seen
where the lines forming the circle touch to complete the circle, but
being only a single pixel it may be difficult to observe.
Can be unpacked anywhere.
Note: This sample was created with the DX8.1 SDK
AppWizard.
|
rt_Rasterize.zip 1.0 |
rt_Skin |
Visualizes vertex blending used for ``skinning'', or making surface
bend smoothly instead of rigidly. The result of vertex blending is
highly dependent on both the transformations used in the blending and
the distribution of the blend weights across the model. This is a
modified version of the VertexBlend sample in the DX8 SDK. The SDK
sample uses a text mesh and varies the blend weight along the x-axis of
the mesh using a fixed weight distribution and transformation. This
sample extends that to a variety of weight distributions and
transformations allowing you to see how each influences the final
result.
Demonstrates: vertex blending
Can be unpacked anywhere.
"Sinusoidal" weight distribution and "Axis Rotation" are the
settings used by the VertexBlend sample.
Note: This sample was created with the DX8.1 SDK
AppWizard. There are two differences with the 8.0 SDK that you will
find if you attempt to compile the sample.
The first difference is that the 8.1 SDK uses a different registry
key to identify the location of the media files. You can adjust the
registry key path in dxutil.cpp in the function
DXUtil_GetDXSDKMediaPath . The second difference is that
in the 8.1 SDK the function D3DXComputeNormals now accepts
an adjacency argument. In d3dfile.cpp on lines 201 and
203, remove the NULL arguments to the function if you are
using the 8.0 SDK.
|
rt_Skin.zip 1.0 |
rt_Text |
Demonstrates drawing text with D3DX. Screen-space text is drawn
using ID3DXFont and CD3DFont. World space text is drawn as a mesh
created as a 3D extruded character outline with D3DXCreateText.
The menus let you change what text is displayed each frame, alter
the color of the displayed text, and select each method's font.
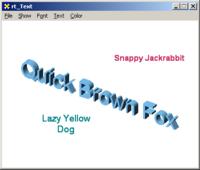
Demonstrates: ID3DXFont, D3DXCreateText, CD3DFont
Unpack into %DX8SDK%\samples\multimedia\Direct3D.
Try CD3DFont with an italic font and watch texels from leak across
glyph boundaries.
|
rt_Text.zip 1.1 |
These samples were written with either the DirectX 8.0 or 8.1 SDK.
If you want to use them with the DirectX 9 SDK, the 8.0 samples might
need slight adjustments to a few functions here and there. The 8.1
samples should compile just fine with the DirectX 9 SDK, although they
will use the 8.1 interfaces and not the 9.0 interfaces.
All of this code assumes that you're using Microsoft Visual C++
6SP4 or Microsoft Visual Studio.Net.
My personal development environment is Windows XP Professional and
Visual Studio.Net 2002.
However, the code should build fine using ANSI settings on Windows
9x/Me. I use the TCHAR convention to avoid strings specific to ANSI
or UNICODE. Any executable included is an ANSI build so you can try
out programs on any of the platforms. (They aren't string intensive
anyway.) You may need to modify the project configurations to
compile in another environment.
If for some reason the supplied executable does not work, try
recompiling the executable from the supplied source. I have gotten
some reports about the executables failing on some systems but I
haven't been able to reproduce this.
Most of these samples require the DX8 Direct3D sample framework in
order to compile. Make sure you unpack them into the
samples\multimedia\Direct3D directory in your DX8 SDK. I've prefixed
their names with 'rt_' so you can easily distinguish them from the
samples that ship with the DX8 SDK.
- Paul Heckbert's zoom for all
your filtering, resampling, resizing and mipmap generation preprocessing
needs. All the thumbnails on this page were created with zoom.
- Windows Installer Tools for
creating simple MSI based installations.
- ImageMagick for dealing
with image file formats not handled by D3DX.
|